Module 5: Managing and Updating Applications with GitOps
Module Overview
This module focuses on managing and updating applications using GitOps practices. It covers making changes through Git, automated updates with Argo CD, rollbacks, and version control. Participants will also have a hands-on exercise to update the Todo app and practice performing rollbacks using Git and Argo CD.
Key Learning Objectives
-
Understand how to make changes to applications through Git.
-
Learn how Argo CD automates updates in OpenShift.
-
Explore rollbacks and version control in GitOps.
-
Practice updating and rolling back the Todo app using Git and Argo CD.
Making Changes through Git
Updating Application Configuration
To update the application, make changes to the configuration files in the Git repository. Follow these steps:
-
Clone the Git repository:
git clone <repository_url>
-
Navigate to the application configuration directory:
cd <repository_directory>/config
-
Make the necessary changes to the configuration files.
-
Commit and push the changes to the Git repository:
git add .
git commit -m "Update application configuration"
git push origin main
Automated Updates with Argo CD
How Argo CD Works
Argo CD automatically detects changes in the Git repository and deploys the updates to OpenShift. Here’s how it works:
-
Argo CD continuously monitors the Git repository for changes.
-
When a change is detected, Argo CD synchronizes the desired state defined in the Git repository with the actual state in OpenShift.
-
The application is automatically updated with the new configuration.
Rollbacks and Version Control
Facilitating Rollbacks
GitOps makes it easy to perform rollbacks and manage version control for application deployments. Here’s how:
-
Identify the commit hash of the desired version to rollback to.
-
Update the Git repository to the desired commit:
git checkout <commit_hash>
git push origin main
-
Argo CD will detect the change and rollback the application to the specified version.
Hands-on Exercise: Managing and Updating the Todo App
Exercise Objectives
-
Update the Todo app by making changes to the Git repository.
-
Observe how Argo CD automatically deploys the updates.
-
Practice performing rollbacks using Git and Argo CD.
Script Overview
This Bash script sets up an ArgoCD environment by creating a secret and a config map in the specified namespace. It also ensures that the necessary policies and roles are in place for the pipeline to function correctly.
Prerequisites
Before running the script, ensure the following:
- You have kubectl
and oc
(OpenShift CLI) installed and configured.
- You have the necessary permissions to create secrets, config maps, and manage policies in the OpenShift cluster.
- The OpenShift cluster is running and accessible.
2. Define Variables
# Define the namespace, secret name, and config map name
USERNAME=yourusername
NAMESPACE="todo-demo-app-${USERNAME}"
SECRET_NAME="argocd-env-secret"
CONFIG_MAP_NAME="argocd-env-configmap"
nameSpace=openshift-gitops
SERVER=$(oc get route openshift-gitops-server -n ${nameSpace} -o jsonpath='{.spec.host}')
USERNAME=admin
PASSWORD="ASK the LAB instructor for password"
-
Purpose:
-
NAMESPACE: The namespace where the secret and config map will be created.
-
SECRET_NAME: The name of the secret to be created.
-
CONFIG_MAP_NAME: The name of the config map to be created.
-
nameSpace: The namespace where the OpenShift GitOps server is running.
-
SERVER: The hostname of the OpenShift GitOps server.
-
USERNAME: The username for ArgoCD.
-
PASSWORD: The password for ArgoCD, retrieved from the OpenShift secret and decoded from base64.
Encode Username and Password
# Encode the username and password as base64
USERNAME_BASE64=$(echo -n "${USERNAME}" | base64)
PASSWORD_BASE64=$(echo -n "${PASSWORD}" | base64)
-
Purpose:
-
USERNAME_BASE64: The username is encoded to base64.
-
PASSWORD_BASE64: The password is encoded to base64.
Create ArgoCD Secret
# Create the ArgoCD secret
oc create secret generic "${SECRET_NAME}" \
--namespace="${NAMESPACE}" \
--from-literal="ARGOCD_USERNAME=$(echo ${USERNAME_BASE64} | base64 --decode)" \
--from-literal="ARGOCD_PASSWORD=$(echo ${PASSWORD_BASE64} | base64 --decode)"
-
Purpose:
-
This command creates a generic Kubernetes secret in the specified namespace.
-
The secret contains the ArgoCD username and password, which are decoded from the base64-encoded values.
Create ArgoCD Config Map
# Create the ArgoCD config map
oc create configmap "${CONFIG_MAP_NAME}" \
--namespace="${NAMESPACE}" \
--from-literal="ARGOCD_SERVER=${SERVER}:443"
-
Purpose:
-
This command creates a Kubernetes config map in the specified namespace.
-
The config map contains the ArgoCD server URL, which includes the hostname and port (443).
Edit pipeline Pipelines→Pipelines→argocd-quay-todo-demo-app-pipeline-yourusername
Update argocd-quay-todo-demo-app-pipeline-yourusername
change todo-demo-app to todo-demo-app-yourusername
|
- name: argocd-task-sync-and-wait
params:
- name: application-name
value: todo-demo-app-yourusername
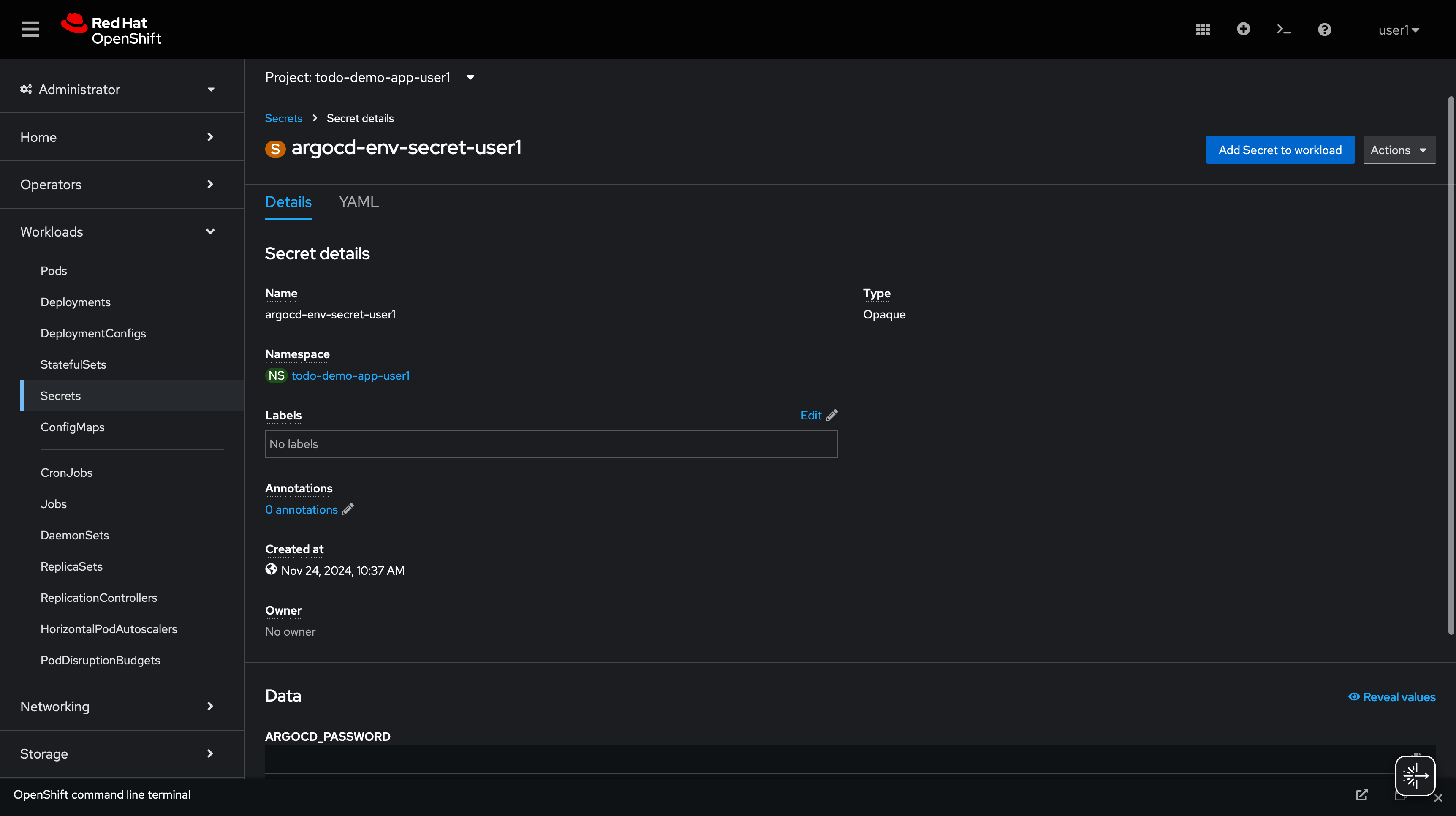
Change the IMAGE_TAG and QUAY_IO_IMAGE_TAG_NAME so there is a change |
Rerun the pipeline
export USERNAME=yourusername
export IMAGE_TAG="v0.0.3"
export QUAY_IO_IMAGE_TAG_NAME="v0.0.3"
export CURRENT_IMAGE_TAG="v1"
export GIT_TOKEN="password"
export QUAY_ROUTE=$(oc get route -n quay-registry | grep quay-registry-quay-quay-registry | awk '{print $2}')
export GITEA_ROUTE=$(oc get route -n gitea | grep gitea | awk '{print $2}')
export QUAY_IO_REPOSITORY="${QUAY_ROUTE}/${USERNAME}/todo-demo-app-${USERNAME}"
export GIT_REPOSITORY="${GITEA_ROUTE}/${USERNAME}/todo-demo-app-helmrepo"
export GIT_EMAIL="pipeline@example.com"
export GIT_NAME="todo-demo-app"
export REPLICA_COUNT="1"
export GIT_USERNAME="${USERNAME}"
export SHARED_WORKSPACE="shared-workspace-pvc-${USERNAME}"
export MAVEN_SETTINGS_WORKSPACE="maven-settings-pvc-${USERNAME}"
export HELM_SHARED_WORKSPACE="helm-workspace-pvc-${USERNAME}"
export SECRET_VOLUME="container-registry-secret-${USERNAME}"
export ARGOCD_SECRET_VOLUME=argocd-env-secret
tkn pipeline start argocd-quay-todo-demo-app-pipeline-${USERNAME} \
--param IMAGE_TAG="${IMAGE_TAG}" \
--param CURRENT_IMAGE_TAG="${CURRENT_IMAGE_TAG}" \
--param quay-io-repository="${QUAY_IO_REPOSITORY}" \
--param quay-io-image-tag-name="${QUAY_IO_IMAGE_TAG_NAME}" \
--param GIT_REPOSITORY="${GIT_REPOSITORY}" \
--param GIT_EMAIL="${GIT_EMAIL}" \
--param GIT_NAME="${GIT_NAME}" \
--param REPLICA_COUNT="${REPLICA_COUNT}" \
--param GIT_USERNAME="${GIT_USERNAME}" \
--param GIT_TOKEN="${GIT_TOKEN}" \
--workspace name=shared-workspace-${USERNAME},claimName="${SHARED_WORKSPACE}" \
--workspace name=maven-settings-${USERNAME},claimName="${MAVEN_SETTINGS_WORKSPACE}" \
--workspace name=helm-shared-workspace-${USERNAME},claimName="${HELM_SHARED_WORKSPACE}" \
--workspace name=secret-volume,secret="${SECRET_VOLUME}" \
--workspace name=argocd-env-secret,secret="${ARGOCD_SECRET_VOLUME}" \
--showlog
What is this Task?
This Task is designed to help you sync (deploy) an Argo CD application and wait for it to be healthy. An Argo CD application is a way to manage and deploy your applications on Kubernetes. Think of it like a pipeline that connects your code in a Git repository to a Kubernetes cluster.
How Do I Use This Task?
To use this Task, you’ll need to provide some information about your Argo CD application and your cluster. Here’s what you’ll need to know:
Application Information
-
Application Name: This is the name of the Argo CD application you want to deploy. This is like the name of your project.
-
Revision: This is the version of your application that you want to deploy. You can think of it like a Git branch or a specific version of your code.
-
Additional Flags: You can add any additional flags that you want to pass to the
argocd app sync
command. For example, you might want to use--force
to override any existing deployments.
Cluster Information
-
Argo CD Server: This is the address of your Argo CD server. This is where your application will be deployed.
-
Username and Password: You’ll need to authenticate with your Argo CD server using a username and password. Make sure you have these credentials set up and ready to go!
-
Authentication Token: If you’re using an authentication token, you can provide that instead of a username and password.
Parameters
The Task has five parameters that you need to fill in:
application-name
-
Type: String
-
Description: The name of the application to deploy.
-
Default Value: None
-
Example Value:
my-application
revision
-
Type: String
-
Description: The revision to deploy.
-
Default Value:
HEAD
(which means the latest version) -
Example Value:
main
orbranch-name
Environment Variables
The Task uses environment variables to connect to your Argo CD server and authenticate. You’ll need to set up the following variables:
Steps
The Task has a single step:
login
-
Image: quay.io/argoproj/argocd:$(params.argocd-version)
-
Name: login
-
Resources: None
-
Script:
if [ -z "$ARGOCD_AUTH_TOKEN" ]; then
yes | argocd login "$ARGOCD_SERVER" --username="$ARGOCD_USERNAME" --password="$ARGOCD_PASSWORD";
fi
argocd app sync "$(params.application-name)" --revision "$(params.revision)" "$(params.flags)"
argocd app wait "$(params.application-name)" --health "$(params.flags)"